The template field. This is where you define how the generated file should look.
Example of a simple xml format.
<?xml version="1.0" encoding="utf-8"?>
<Invoice>
<InvoiceNumber>@Get('DocNum')</InvoiceNumber>
<CustomerCode>@Get('CardCode')</CustomerCode>
<CustomerName>@Get('CardName')</CustomerName>
<InvoiceLines>
#foreach(@line in @DocumentLines)
#BEGIN
<Line Item="@line.Get('ItemCode')" Quantity="@line.Get('Quantity')"/>
#END
</InvoiceLines>
</Invoice>
And its result (given invoice is number 373 to Earthshaker Corporation)
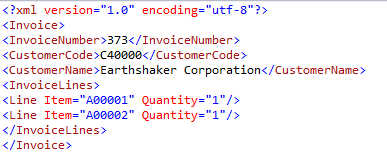
In this case we have a very simple xml format but it could be any kind of format.
The @Get('x') are the keywords that are going to be replaced with header data.
The @x.Get('x') is the syntax for inserting line data.
The syntax for looping trough line data and inserting data is:
#foreach(@xLine in @xLines)
#BEGIN
<Tag Id="@xLine.Get('x')" Value="@xLine.Get('x')"/>
#END
NOTE: If you select XML as file type UFFE will automatically XML encode strings when using @Get('x')
NOTE: You are also able to access header data in a foreach loop by using the header syntax.
NOTE: You can substring a value by using the syntax: @Get('x',5) where 5 is the max length of the string. Example @Get('CardName',30) would only give you the first 30 characters of the CardName.
NOTE: You can use the keyword @GetRaw('x') to get the raw value and you can use @GetXmlEncoded('x') to get the an XML encoded string. They also accept a second parameter for substring.
NOTE: You can use the keyword $[TAB] to create at txt Tabulator and $[NEWLINE] to create a line break if needed.
NOTE: You have the option to use IF/ELSEIF/ELSE in your UFFE. Example:
#foreach(@bp in @BusinessPartners)
#BEGIN
#IF(@bp.Get('CardCode') = 'C20000')
#BEGIN
<BusinessPartnerSpecial>@bp.Get('CardName')</BusinessPartnerSpecial>
#END
#ELSEIF(@bp.Get('CardCode') = 'C23900')
#BEGIN
<BusinessPartnerSpecial2>@bp.Get('CardName')</BusinessPartnerSpecial2>
#END
#ELSE
#BEGIN
<BusinessPartner>@bp.Get('CardName')</BusinessPartner>
#END
#END
Warning: You are not allowed to use parenthesis in the columns names of the SQL result. This will break the .Get() syntax.
|